mirror of
https://github.com/luau-lang/luau.git
synced 2024-12-13 21:40:43 +00:00
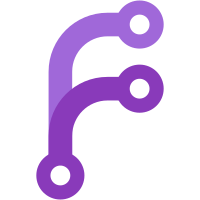
### What's changed? * Syntax for [read-only and write-only properties](https://github.com/luau-lang/rfcs/pull/15) is now parsed, but is not yet supported in typechecking ### New Type Solver * `keyof` and `rawkeyof` type operators have been updated to match final text of the [RFC](https://github.com/luau-lang/rfcs/pull/16) * Fixed issues with cyclic type families that were generated for mutable loop variables ### Native Code Generation * Fixed inference for number / vector operation that caused an unnecessary VM assist --- ### Internal Contributors Co-authored-by: Aaron Weiss <aaronweiss@roblox.com> Co-authored-by: Andy Friesen <afriesen@roblox.com> Co-authored-by: Lily Brown <lbrown@roblox.com> Co-authored-by: Vyacheslav Egorov <vegorov@roblox.com>
62 lines
1.4 KiB
C++
62 lines
1.4 KiB
C++
// This file is part of the Luau programming language and is licensed under MIT License; see LICENSE.txt for details
|
|
// This code is based on Lua 5.x implementation licensed under MIT License; see lua_LICENSE.txt for details
|
|
#include "lua.h"
|
|
|
|
#ifdef _WIN32
|
|
#ifndef WIN32_LEAN_AND_MEAN
|
|
#define WIN32_LEAN_AND_MEAN
|
|
#endif
|
|
#ifndef NOMINMAX
|
|
#define NOMINMAX
|
|
#endif
|
|
#include <windows.h>
|
|
#endif
|
|
|
|
#ifdef __APPLE__
|
|
#include <mach/mach.h>
|
|
#include <mach/mach_time.h>
|
|
#endif
|
|
|
|
|
|
#include <time.h>
|
|
|
|
static double clock_period()
|
|
{
|
|
#if defined(_WIN32)
|
|
LARGE_INTEGER result = {};
|
|
QueryPerformanceFrequency(&result);
|
|
return 1.0 / double(result.QuadPart);
|
|
#elif defined(__APPLE__)
|
|
mach_timebase_info_data_t result = {};
|
|
mach_timebase_info(&result);
|
|
return double(result.numer) / double(result.denom) * 1e-9;
|
|
#elif defined(__linux__)
|
|
return 1e-9;
|
|
#else
|
|
return 1.0 / double(CLOCKS_PER_SEC);
|
|
#endif
|
|
}
|
|
|
|
static double clock_timestamp()
|
|
{
|
|
#if defined(_WIN32)
|
|
LARGE_INTEGER result = {};
|
|
QueryPerformanceCounter(&result);
|
|
return double(result.QuadPart);
|
|
#elif defined(__APPLE__)
|
|
return double(mach_absolute_time());
|
|
#elif defined(__linux__)
|
|
timespec now;
|
|
clock_gettime(CLOCK_MONOTONIC, &now);
|
|
return now.tv_sec * 1e9 + now.tv_nsec;
|
|
#else
|
|
return double(clock());
|
|
#endif
|
|
}
|
|
|
|
double lua_clock()
|
|
{
|
|
static double period = clock_period();
|
|
|
|
return clock_timestamp() * period;
|
|
}
|