mirror of
https://github.com/luau-lang/luau.git
synced 2025-01-20 01:38:07 +00:00
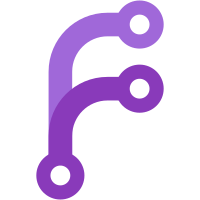
All of our changes this week have been focused on the new type solver and the JIT. As we march toward feature parity with the old solver, we've tightened up a bunch of lingering issues with overload resolution, unsealed tables, and type normalization. We've also fixed a bunch of crashes and assertion failures in the new solver. On the JIT front, we've started work on an A64 backend, improved the IR analysis in a bunch of cases, and implemented assembly generation for the builtin functions `type()` and `typeof()`. --------- Co-authored-by: Arseny Kapoulkine <arseny.kapoulkine@gmail.com> Co-authored-by: Vyacheslav Egorov <vegorov@roblox.com>
89 lines
1.4 KiB
C++
89 lines
1.4 KiB
C++
// This file is part of the Luau programming language and is licensed under MIT License; see LICENSE.txt for details
|
|
#include "Luau/Unifiable.h"
|
|
|
|
namespace Luau
|
|
{
|
|
namespace Unifiable
|
|
{
|
|
|
|
static int nextIndex = 0;
|
|
|
|
int freshIndex()
|
|
{
|
|
return ++nextIndex;
|
|
}
|
|
|
|
Free::Free(TypeLevel level)
|
|
: index(++nextIndex)
|
|
, level(level)
|
|
{
|
|
}
|
|
|
|
Free::Free(Scope* scope)
|
|
: index(++nextIndex)
|
|
, scope(scope)
|
|
{
|
|
}
|
|
|
|
Free::Free(Scope* scope, TypeLevel level)
|
|
: index(++nextIndex)
|
|
, level(level)
|
|
, scope(scope)
|
|
{
|
|
}
|
|
|
|
int Free::DEPRECATED_nextIndex = 0;
|
|
|
|
Generic::Generic()
|
|
: index(++nextIndex)
|
|
, name("g" + std::to_string(index))
|
|
{
|
|
}
|
|
|
|
Generic::Generic(TypeLevel level)
|
|
: index(++nextIndex)
|
|
, level(level)
|
|
, name("g" + std::to_string(index))
|
|
{
|
|
}
|
|
|
|
Generic::Generic(const Name& name)
|
|
: index(++nextIndex)
|
|
, name(name)
|
|
, explicitName(true)
|
|
{
|
|
}
|
|
|
|
Generic::Generic(Scope* scope)
|
|
: index(++nextIndex)
|
|
, scope(scope)
|
|
{
|
|
}
|
|
|
|
Generic::Generic(TypeLevel level, const Name& name)
|
|
: index(++nextIndex)
|
|
, level(level)
|
|
, name(name)
|
|
, explicitName(true)
|
|
{
|
|
}
|
|
|
|
Generic::Generic(Scope* scope, const Name& name)
|
|
: index(++nextIndex)
|
|
, scope(scope)
|
|
, name(name)
|
|
, explicitName(true)
|
|
{
|
|
}
|
|
|
|
int Generic::DEPRECATED_nextIndex = 0;
|
|
|
|
Error::Error()
|
|
: index(++nextIndex)
|
|
{
|
|
}
|
|
|
|
int Error::nextIndex = 0;
|
|
|
|
} // namespace Unifiable
|
|
} // namespace Luau
|