mirror of
https://github.com/luau-lang/luau.git
synced 2025-01-08 04:19:09 +00:00
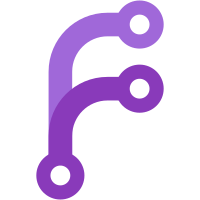
# What's Changed? - Code refactoring with a new clang-format - More bug fixes / test case fixes in the new solver ## New Solver - More precise telemetry collection of `any` types - Simplification of two completely disjoint tables combines them into a single table that inherits all properties / indexers - Refining a `never & <anything>` does not produce type family types nor constraints - Silence "inference failed to complete" error when it is the only error reported --- ### Internal Contributors Co-authored-by: Aaron Weiss <aaronweiss@roblox.com> Co-authored-by: Andy Friesen <afriesen@roblox.com> Co-authored-by: Dibri Nsofor <dnsofor@roblox.com> Co-authored-by: Jeremy Yoo <jyoo@roblox.com> Co-authored-by: Vighnesh Vijay <vvijay@roblox.com> Co-authored-by: Vyacheslav Egorov <vegorov@roblox.com> --------- Co-authored-by: Aaron Weiss <aaronweiss@roblox.com> Co-authored-by: Alexander McCord <amccord@roblox.com> Co-authored-by: Andy Friesen <afriesen@roblox.com> Co-authored-by: Vighnesh <vvijay@roblox.com> Co-authored-by: Aviral Goel <agoel@roblox.com> Co-authored-by: David Cope <dcope@roblox.com> Co-authored-by: Lily Brown <lbrown@roblox.com> Co-authored-by: Vyacheslav Egorov <vegorov@roblox.com>
42 lines
1.4 KiB
C++
42 lines
1.4 KiB
C++
// This file is part of the Luau programming language and is licensed under MIT License; see LICENSE.txt for details
|
|
#pragma once
|
|
|
|
#include "Luau/Ast.h"
|
|
#include "Luau/Bytecode.h"
|
|
#include "Luau/DenseHash.h"
|
|
#include "ValueTracking.h"
|
|
|
|
#include <string>
|
|
|
|
namespace Luau
|
|
{
|
|
class BytecodeBuilder;
|
|
|
|
struct BuiltinAstTypes
|
|
{
|
|
BuiltinAstTypes(const char* vectorType)
|
|
: vectorType{{}, std::nullopt, AstName{vectorType}, std::nullopt, {}}
|
|
{
|
|
}
|
|
|
|
// AstName use here will not match the AstNameTable, but the was we use them here always force a full string compare
|
|
AstTypeReference booleanType{{}, std::nullopt, AstName{"boolean"}, std::nullopt, {}};
|
|
AstTypeReference numberType{{}, std::nullopt, AstName{"number"}, std::nullopt, {}};
|
|
AstTypeReference stringType{{}, std::nullopt, AstName{"string"}, std::nullopt, {}};
|
|
AstTypeReference vectorType;
|
|
};
|
|
|
|
void buildTypeMap(
|
|
DenseHashMap<AstExprFunction*, std::string>& functionTypes,
|
|
DenseHashMap<AstLocal*, LuauBytecodeType>& localTypes,
|
|
DenseHashMap<AstExpr*, LuauBytecodeType>& exprTypes,
|
|
AstNode* root,
|
|
const char* vectorType,
|
|
const DenseHashMap<AstName, uint8_t>& userdataTypes,
|
|
const BuiltinAstTypes& builtinTypes,
|
|
const DenseHashMap<AstExprCall*, int>& builtinCalls,
|
|
const DenseHashMap<AstName, Compile::Global>& globals,
|
|
BytecodeBuilder& bytecode
|
|
);
|
|
|
|
} // namespace Luau
|